Objectives
- Use CSS Grid layout and CSS Flexbox to style contents
- Learn how to use important React features:
useState
,useEffect
, event handling - Apply various techniques of using CSS styles in an HTML-based web "application"
Overview
This assignment is a redo of the previous Wordle Clone Assignment but implemented as React Functional components. You are not required to incorporate Firebase Authentication or Firestore into this React version.
TIP
Be sure you use React functional components and NOT React class-based components. You may work with another classmate on this programming assignment.
Project Setup
Accept your instructor GitHub classroom invitation to initialize a GitHub repo under your GitHub account.
IMPORTANT
If you work in a group of two, the grade of each member will be determined from the member contribution to the project as reported by
git
statistics.Clone the repo just created to your local computer so you can making changes
Installed the required packages by typing (depending on your computer and Internet connection this process may take a while)
bashnpm install
Run a local development server (on default port 5173)
bashnpm run dev
Use your browser to open
localhost:5173
(or use the port number as instructed by the above command). You will find the main page with two functional buttons "Add Hello" and "Remove All"
The generated project includes the following starter files/directories:
Top-level Project
├── src
│ ├── App.css
│ ├── App.tsx
│ ├── index.css
│ ├── main.tsx
│ ├── vite-env.d.ts
│ └── Wordle.tsx
│
├── index.html
├── package.json
├── tsconfig.json
├── tsconfig.node.json
└── vite.config.ts
Wordle Implementation Requirements
The following screenshot is provided for your reference so the requirements specified below make sense.
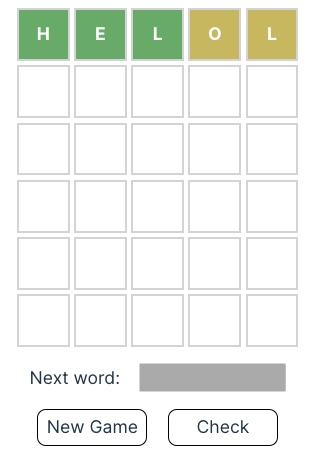
The following are minimal requirements to earn a full credit on this assignment. The order presented below should not be used as a hard reference for your workflow in developing the application. I strongly recommend you work the details of the game logic before tackling the user interface presentation and styling details.
Show your name(s) somewhere on the page
Show a grid of 6 rows by 5 columns to display the letters of each word. Each cell in the grid should be displayed using a uniform size. Use CSS grid to style this grid.
Provide a button ("New Game") to secretly select a random 5-letter word. When a previous session of the game is already running, this button will also clear the grid
IMPORTANT
For ease of grading by your instructor, provide a mechanism to reveal the secret word on the UI. Either using a button, checkbox, or key press combination.
On the grid, each letter must be displayed in uppercase and centered within its cell (both horizontally and vertically). Hint: consider using CSS Flex box to achieve this visual effect
Provide an input box for the user to type his next word guess and a button for the user to have the current word entered and checked. In addition, pressing this button should clear the current word in the input field.
TIP
The NY Times version updates the grid on each keystroke. Your implementation is not required to have this feature, but it will be an extra credit (see below)
When displayed on the grid each letter should be displayed with three different background colors:
- Green for letters which appear in the secret word in the correct spot
- Yellow for letters which appear in the secret word but in the the wrong spot
- Gray for letters which do not appear in the secret word
Disable the "Check" button when the user correctly guess the secret word or it has reached six attempts without guessing the secret word.
Show some type of "congratulation" message when the user is able to guess the secret word or "game over" message otherwise.
On the same page of your Wordle game add a "brief report" explaining the details of your React TSX code for building the grid of letters
Checking Matching Letters
Checking the matches of two words with no duplicate letters is straightforward. However when one of the words has duplicate letters, it may require extra explanation.
Secret Word: DOLLY
Guess Word : BUDDY
should count as one perfect match (Y) and one misplaced match (D). A flawed algorithm may incorrectly report one perfect match (Y) and two misplaced matches (D). If your previous implementation failed to handle the duplicate letter case correctly, you can adapt the following code into your implementation:
function verifyMatch (secret: string, guess: string) {
const matched = [false, false, false, false, false]
const outcome = [".", ".", ".", ".", "."]
let perfectMatchCount = 0
for (let k = 0; k < 5; k++) {
if (guess.charAt(k) == secret.charAt(k)) {
matched[k] = true
outcome[k] = "P" // perfect match
perfectMatchCount++
}
}
if (perfectMatchCount < 5) {
for (let g = 0; g < 5; g++) {
const guessLetter = guess.charAt(g)
let hasMatch = false
for (let s = 0; s < 5; s++) {
if (s == g) continue // don't do perfect match
if (matched[s]) continue
if (outcome[g] != '.') {
hasMatch = true
continue
}
const secretLetter = secret.charAt(s)
if (secretLetter == guessLetter) {
outcome[g] = "M" // misplaced match
hasMatch = true
matched[s] = true
break
}
}
if (!hasMatch) outcome[g] = "N" // no match
}
}
// Array outcome should encode the matching results
}
IMPORTANT
In the list of curated secret words, you must include 5-letter words containing duplicate letters, such as: "FLUFF", "CANNY", "SPOON", etc.
Useful Hints
You may be tempted to use a 2D array to present the words on the grid. I personally would not take this approach. In my opinion, it is much easier to treat each individual word as a string and use the
charAt()
method (similar to Java) to pull each letter of the word when displaying them on the grid.Use CSS classes to render individual letter in different background. For instance, the first row in the sample screenshot above can be reproduced using the following HTML:
html<span class="perfect">H</span> <span class="perfect">E</span> <span class="perfect">L</span> <span class="misplaced">O</span> <span class="misplaced">L</span>
in conjunction with the following CSS:
css.perfect { background: green; /* some other style properties here */ } .misplaced { background: yellow; /* some other style properties here */ }
In case you need to apply multiple styles on an element, CSS allows use of multiple classes:
html<span class="perfect box">G</span> <span class="box perfect">H</span> <!-- order independent -->
in conjunction with the following CSS:
css.box { /* style definitions here */ }
Grading Rubrics
Grading Item | Point |
---|---|
Random selection of secret word | 3 |
Handling user input | 3 |
Handling the "Check" button | 3 |
Handling the "New Game" button | 3 |
Cells in the grid shown in uniform size | 3 |
Empty grid setup (without words) | 3 |
Grid showing words and color-coded matching results | 6 |
Report (included on the page) | 6 |