Password Generator (Android)
Learning Objectives
- Develop familiarity with essential Android concepts
- Develop familiarity with Android Studio features: layout editor, debugger.
- Become more proficient in Kotlin
- Practice scene transitions with Android Intent
- Practice using Activity lifecycle functions
- Practice implementation of the MVVM design pattern using Android ViewModel
Please work in pairs on this homework assignment. I will assume you will self-pair, but if you cannot find somebody to pair with, let me know. Put the name of each student in the header of each Swift source file in the project.
TIP
We will be using GitHub Classroom to manage homework submissions from now on. That won't change a whole lot in terms of how you use Git, it only affects how you will initially create your repo. Instead of creating the repo from scratch under your GitHub account, you will follow the GitHub classroom assignment link to create the repo for your solution.
Important
When creating your Git repository, be sure that the .git
folder is a sibling directory of the app
folder.
Redo the previous assignment for password generation, but this time for Android devices.
Your app will consist of two Android Activity classes:
- The first Android activity (left screenshot below) handles random password generation.
- The second Activity (right screenshot below) is the settings activity to customize password generation details. The Android equivalent of the iOS UISlider is a seek bar.
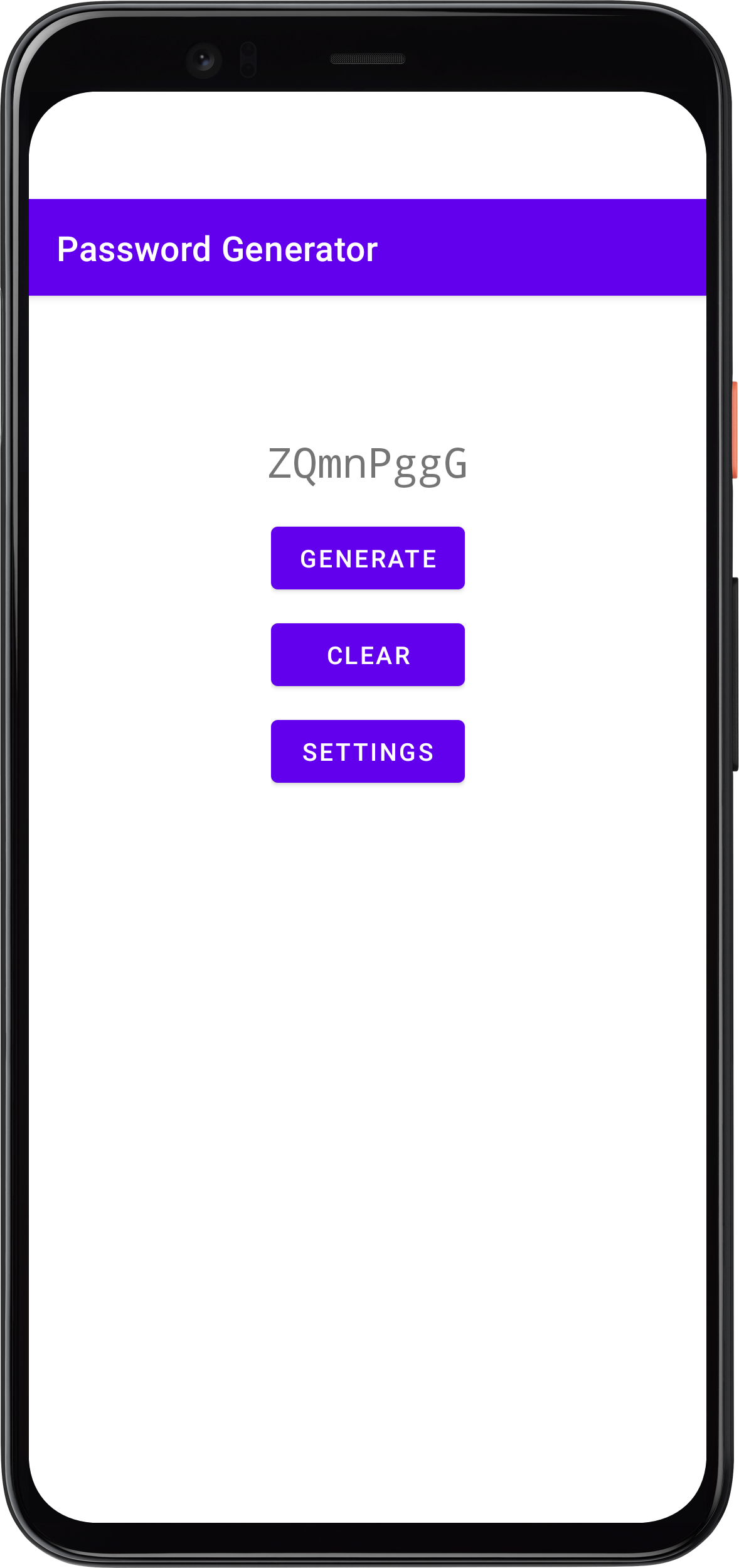
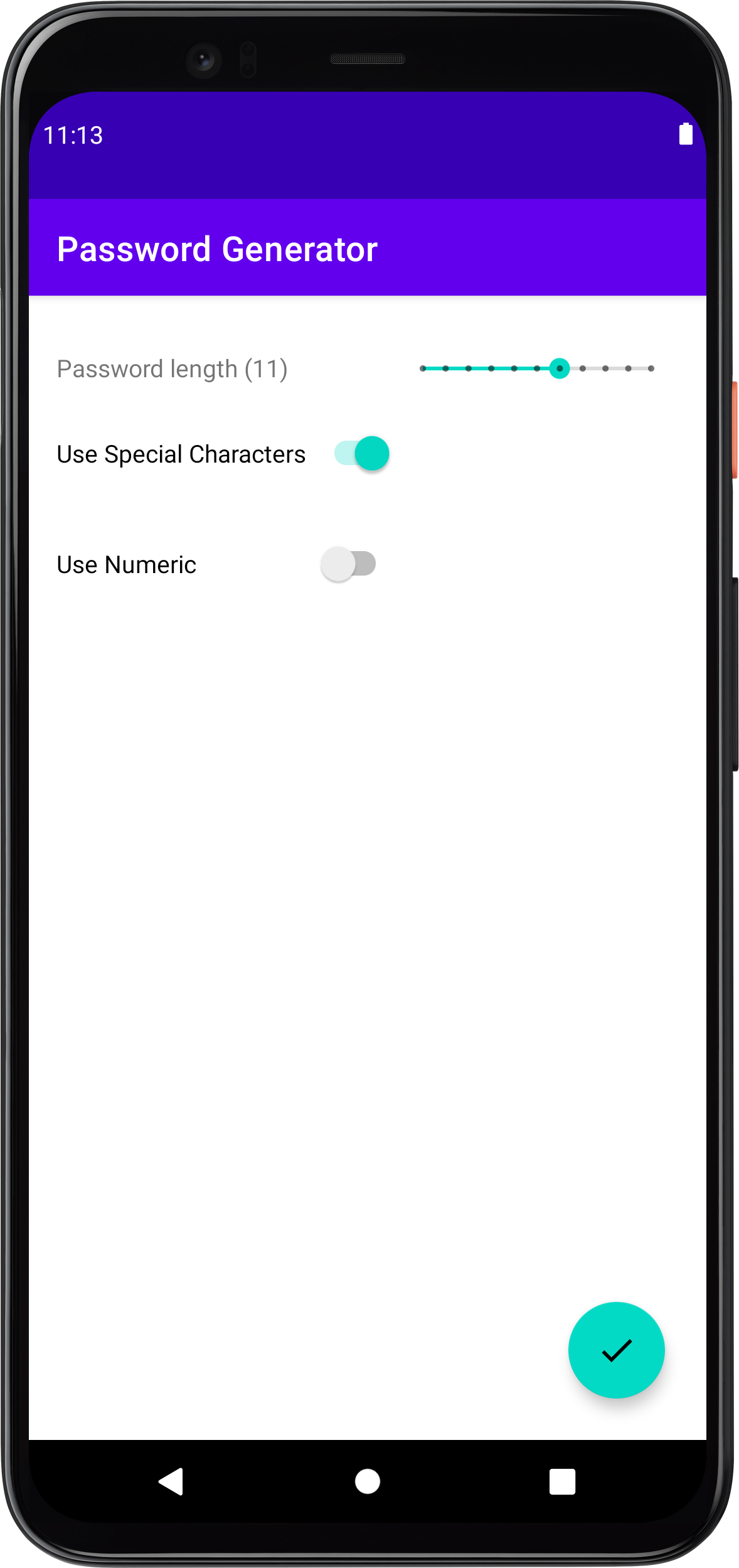
- Place the three buttons on the main screen inside a vertical LinearLayout (Android equivalent of iOS UIStackView)
- The widgets in the settings screen should be initialized with the most recent options selected by the user. Your app is not required to retained these values across multiple launches of the app. When the app is relaunched, it is ok to use the default settings initialized in the main password generation view controller.
Adding ViewModel Classes
Your Activity classes (the "View" in the MVVM design pattern) should neither contain any business logic nor business data. The main responsibility of the View is to update the UI and to handle user input. All the business logic and data should be delegated in the ViewModel.
Landscape Screen Orientation
Viewed in landscape orientation, the main screen should be designed using the following widget arrangment:
- The password should be vertically centered and 40% of the screen width
- The three buttons should be vertically centered as well
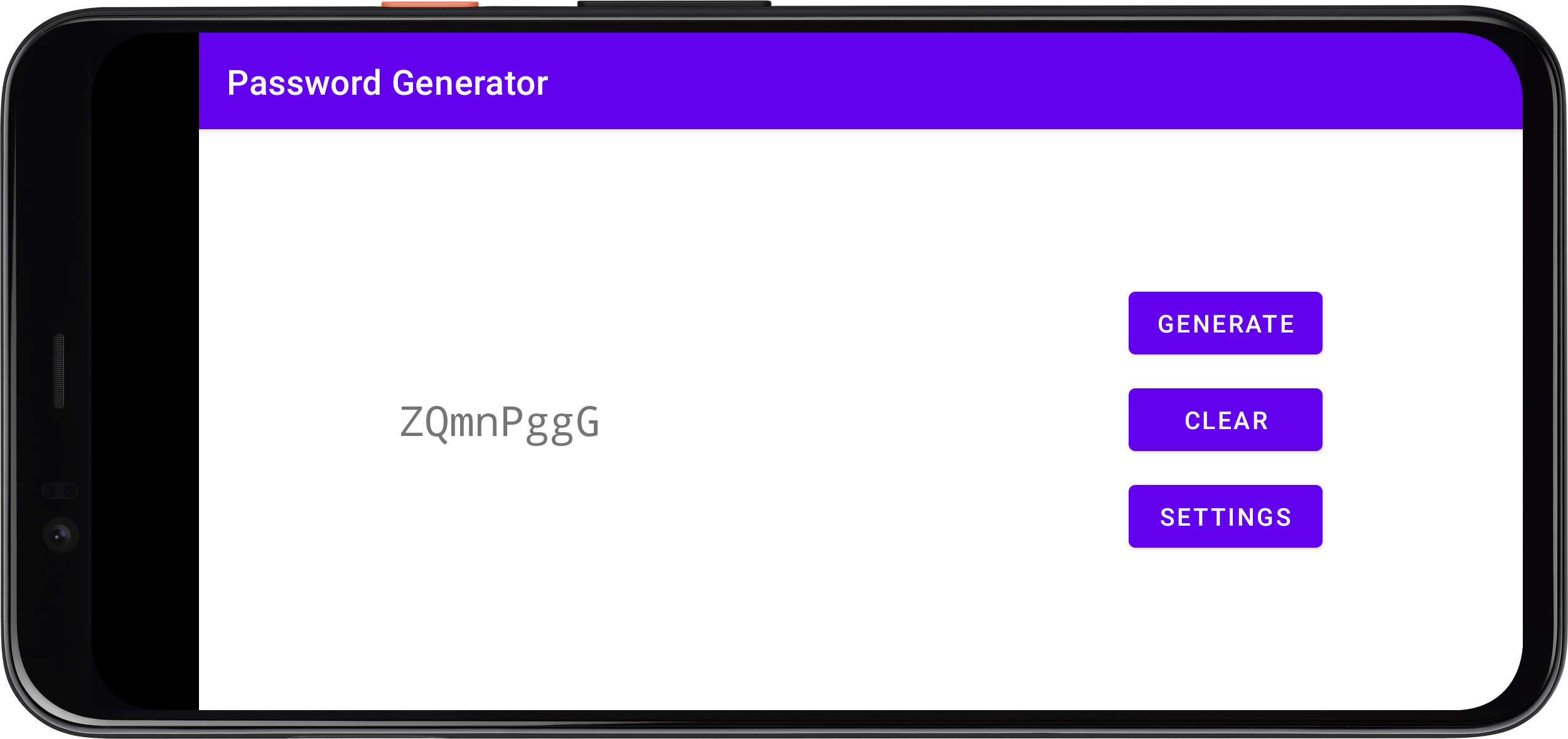
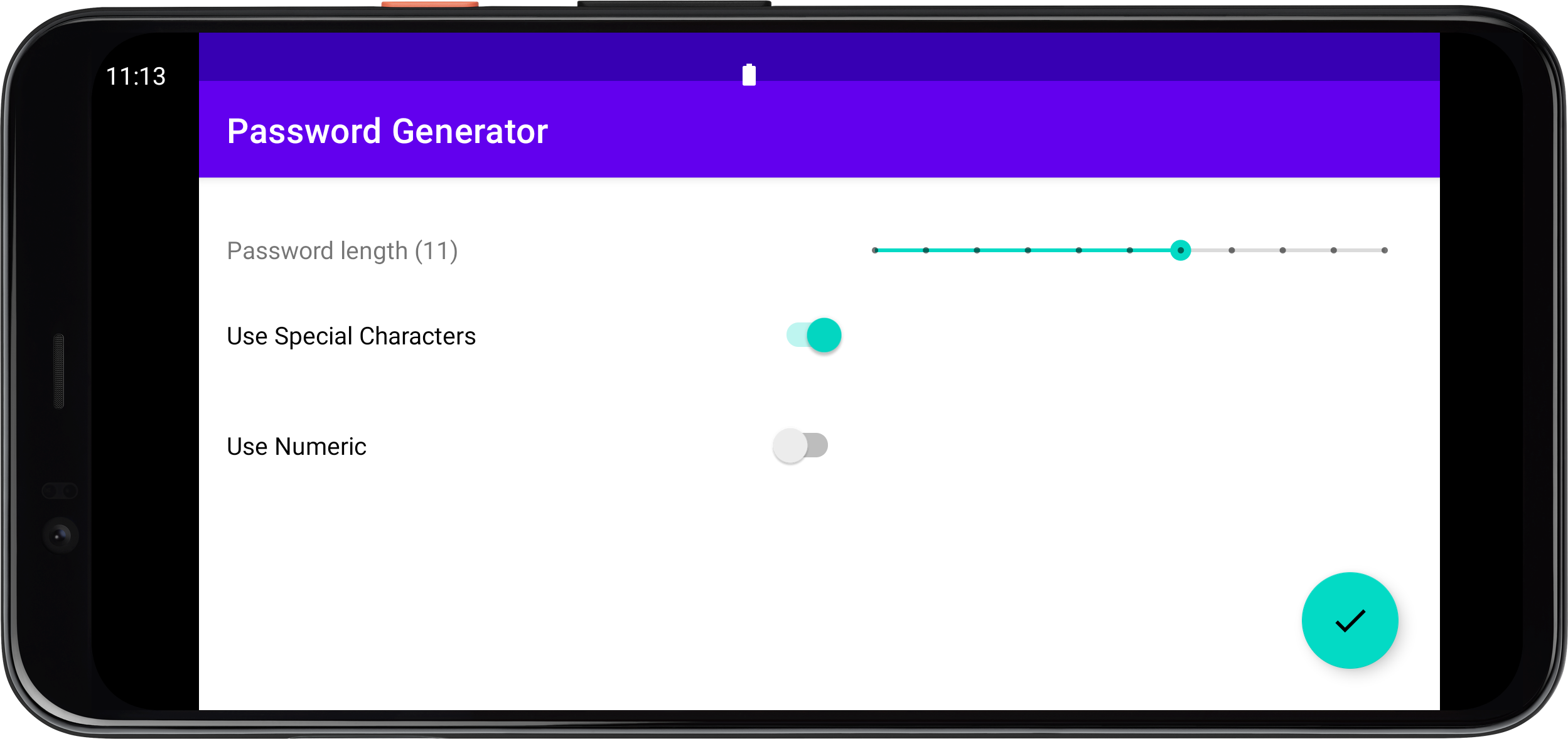
Unlike iOS version where we use two buttons in the top bar, the settings activity in the Android version will show only one Floating Action Button on the lower right corner. This button confirms that the user wants to apply the current settings for generating password and returns to the main screen. When the user press the phone "Back" button (instead of the Action Button above), the settings will not get updated.
In Android you will be using Intent
to pass data forward (from main to setting activity) as well as backward (from setting to main activity).
Deliverables
Please the following very carefully:
Make sure you have git-pushed all of your code updates to the repo you created via this GitHub Classroom invitation link by the due date.
IMPORTANT
The instructor will grade your homework based on the last commit pushed prior to the due date on the default branch (typically the
main
branch) of your repo. If your solution was developed in a different branch, be sure merge it to the main branch or change your default branch setting on GitHub. I will not browse through multiple branches in your repo.There is nothing to submit via Blackboard
Grading Rubrics
Grading Item | Point |
---|---|
Project setup in GitHub | 2 |
Using Android ViewModel to correctly retain data | 4 |
Password Generator using letters only | 2 |
Clear password | 2 |
Design the UI for both portrait and landscape | 4 |
Using Intent to pass data from Password to Settings Activity | 3 |
Using Intent to pass data from Settings to Password Activity | 3 |
Handling slider input | 3 |
Handling toggle switches | 4 |
Handling Action/Back buttons | 3 |
Using special characters in password generation | 2 |
Using numeric characters in password generation | 2 |